Configuration¶
All configuration settings are stored in the configuration object c
, which is structured very similarly to the YAML configuration file,
import dynamite as dyn
c = dyn.config_reader.Configuration('config_file.yaml') # read the configuration fie
######################################################
# config. options relating to the physical system
######################################################
# system_attributes accessed via:
c.system.distMPc # etc
# system_components stores in the list:
c.system.cmp_list
c.system.cmp_list[0] # the 0'th component,
c.system.cmp_list[1] # the 1'st component, etc...
# component parameters are stored in the list:
c.system.cmp_list[0].parameters
# system_parameters are stored in:
c.system.parameters
# all parameters (component and system) are stored together in the list:
c.parspace
######################################################
# config. options relating to other settings
######################################################
# orblib_settings stored in the dictionary:
c.settings.orblib_settings
# weight_solver_settings stored in the dictionary:
c.settings.weight_solver_settings
# multiprocessing_settings stored in the dictionary:
c.settings.multiprocessing_settings
# io_settings stored in the dictionary:
c.settings.io_settings
# legacy_settings stored in the dictionary:
c.settings.legacy_settings
API¶
Classes:
Configuration
([filename, reset_logging, ...])Reads configuration file
DynamiteLogging
([logfile, console_level, ...])Dynamite logging setup.
Settings
()Class to hold all configuration settings
UniqueKeyLoader
(stream)Special yaml loader with duplicate key checking.
- class config_reader.Configuration(filename=None, reset_logging=False, user_logfile='dynamite', reset_existing_output=False)¶
Reads configuration file
Does some rudimentary checks for consistency. Builds the output directory tree if it does not exist already (does not delete existing data).
- Parameters:
- filenamestring
needs to refer to an existing file including path
- reset_loggingbool
if False: use the calling application’s logging settings if True: set logging to Dynamite defaults
- user_logfilestr or None or False
Name of the logfile (
.log
will be appended). Special values:user_logfile=None
will not create a logfile.user_logfile=False
will create a UTC-timestamped logfiledynamiteYYMMDD-HHMMSSuuuuuu.log
. Will be ignored ifreset_logging=False
. The default isuser_logfile='dynamite'
.- reset_existing_outputbool
if False: do not touch existing data in the output directory tree if True: rebuild the output directory tree and delete existing data
- Returns:
- sets attributes:
self.system
: adyn.physical_system.System
object
self.cmp_list
: a list ofdyn.physical_system.Component
objects
self.settings
: a list ofdyn.config_reader.Settings
object
self.settings
: a list ofdyn.config_reader.Settings
object- Raises:
- FileNotFoundError
If file does not exist or filename is None or not given.
Methods:
backup_config_file
([reset, keep, delete_other])Copy the config file to the output directory.
copy_config_file
(dest_directory[, clean])Copy config file to dest_directory.
Get 2 * number of kinematic observations
Create output directory tree.
remove_all_existing_output
([wipe_all, ...])Removes all existing DYNAMITE output.
Deletes the all models file
Removes existing orbital weights ('ml' directories).
Removes the entire model output tree, including all existing orblibs
remove_existing_plots
([remove_directory])Removes existing plots from the plots directory.
set_threshold_del_chi2
(generator_settings)Sets threshold_del_chi2 depending on scaled or unscaled input.
validate
()Validates the system and settings.
validate_chi2
([which_chi2])Validates which_chi2 setting
- backup_config_file(reset=False, keep=None, delete_other=False)¶
Copy the config file to the output directory.
A running index of the format _xxx will be appended to the base file name to keep track of earlier config files (config_000.yaml, config_001.yaml, config_002.yaml, etc…)
This method is not used in standard DYNAMITE and provided as a utility.
- Parameters:
- resetbool, optional
If reset==True, all *.yaml files in the output directory are deleted before the config file is copied. The default is False.
- keepint or NoneType, optional
If an integer > 0, at most keep config files WITH THE SAME BASE NAME are kept. Used to control the number of config file backups in the output folder. Can be combined with delete_other. The current config file will always be backuped. If keep==None, nothing is done. The default is None.
- delete_otherbool, optional
If delete_other==True, all config files WITH A DIFFERENT BASE NAME will be deleted. If delete_other==False, nothing is done. The default is False.
- Returns:
- None.
- copy_config_file(dest_directory, clean=True)¶
Copy config file to dest_directory.
Creates a copy of the config file, intended to add it to the directory holding the model results. The file date will be preserved if possible.
- Parameters:
- dest_directorystr, mandatory
The directory the config file will be copied to.
- cleanbool, optional
If True, all *.yaml files in dest_directory will be deleted before copying. Default is True.
- get_2n_obs()¶
Get 2 * number of kinematic observations
Used for scaling threshold chi2 values for parameter searches. For kinemtic type:
GaussHermite
, then n_obs = number_GH * number_spatial_bins
BayesLOSVD
, then n_obs = n_LOSVD_bins * number_spatial_binsThis returns the sum of (2 * n_obs) for all kinematic sets. Take kinematics from the
TriaxialVisibleComponent
of the system.
- Returns:
- int
2 * total number of kinematic observations
- get_n_cpus()¶
” Returns the number of avalable CPUs.
- make_output_directory_tree()¶
Create output directory tree. Existing directories not affected.
- Returns:
- None.
- remove_all_existing_output(wipe_all=False, create_tree=True)¶
Removes all existing DYNAMITE output.
The options determine whether non-DYNAMITE output shall survive in the output folders. Also resets
self.all_models
to an emptyAllModels
object.
- Parameters:
- wipe_allBool, optional
If True, the complete output directory tree will be removed. Set to False to keep (a) user files & directories in the output directory and (b) user directories in the plots directory. The default is False.
- create_treeBool, optional
If True, recreates an empty output directory tree with a backup of the config file. Does not recreate directories if False. The default is True.
- Returns:
- None.
- Raises:
- Exception if wipe_all==True and the output directory tree cannot
- be deleted.
- remove_existing_all_models_file(wipe_other_files=False)¶
Deletes the all models file
Deletes the all models file if it exists and optionally removes all other regular files in the output directory. Additionally resets
self.all_models
to an emptyAllModels
object.
- Parameters:
- wipe_other_filesBool, optional
If True, all regular files in the output directory will be deleted and a new backup of the config file will be created. If False, only the all models file will be removed. The default is False.
- Returns:
- None.
- Raises:
- Exception if file(s) cannot be removed.
- remove_existing_orbital_weights()¶
Removes existing orbital weights (‘ml’ directories).
Deletes all files matching
output/*/ml*/
- Returns:
- None.
- Raises:
- Exception if directories cannot be removed.
- remove_existing_orblibs()¶
Removes the entire model output tree, including all existing orblibs
- Returns:
- None.
- remove_existing_plots(remove_directory=False)¶
Removes existing plots from the plots directory.
Optionally, the plot directory tree can be removed recursively.
- Parameters:
- remove_directoryBOOL, optional
True if the plot directory shall be removed, too. If False, only regular files in the plot directory are deleted (subdirectories will remain untouched in that case). The default is False.
- Returns:
- None.
- Raises:
- Exception if files/directories cannot be removed.
- set_threshold_del_chi2(generator_settings)¶
Sets threshold_del_chi2 depending on scaled or unscaled input.
Works with the legacy setup only (stars component of class TriaxialVisibleComponent with one or more sets of kinematics).
- Parameters:
- generator_settingsgenerator_settings dict
- Returns:
- None.
- validate()¶
Validates the system and settings.
This method is still VERY rudimentary and will be adjusted as we add new functionality to dynamite. Currently, this method is geared towards legacy mode.
- Returns:
- None.
- validate_chi2(which_chi2=None)¶
Validates which_chi2 setting
Validates the which_chi2 setting in the config file (if argument which_chi2 is None) or the string given in the argument.
- Parameters:
- which_chi2str, optional
If None, the which_chi2 setting in the config file is validated; if not None, the string given is validated. The default is None.
- Returns:
- which_chi2str
The valid which_chi2 setting: either the value from the config file or the string passed as an argument.
- Raises:
- ValueError
If which_chi2 fails validation.
- class config_reader.DynamiteLogging(logfile='dynamite', console_level=20, logfile_level=10, console_formatter=None, logfile_formatter=None)¶
Dynamite logging setup.
ONLY use if logging has not been configured outside of Dynamite. If no arguments are given, the logging setup is as follows:
log to the console with logging level INFO, messages include the level, timestamp, class name, and message text
create a log file with logging level DEBUG, messages include the level, timestamp, class name, filename:method:line number, and message text
- Parameters:
- logfilestr or None or False, optional
Name of the logfile (
.log
will be appended). Special values:logfile=None
will not create a logfile.logfile=False
will create a UTC-timestamped logfiledynamiteYYMMDD-HHMMSSuuuuuu.log
. The default islogfile='dynamite'
.- console_levelint, optional
Logfile logging level. The default is logging.INFO.
- logfile_levelint, optional
Console logging level. The default is logging.DEBUG.
- console_formatterstr, optional
Format string for console logging. The default is set in the code.
- logfile_formatterstr, optional
Format string for logfile logging. The default is set in the code.
- class config_reader.Settings¶
Class to hold all configuration settings
Has a dictionary attribute for each entry of the second section of the YAML config file (e.g. orblib_settings etc…)
Methods:
add
(kind, values)Add each setting to the object
validate
()Validate that all expected settings are present and perform checks
- add(kind, values)¶
Add each setting to the object
- validate()¶
Validate that all expected settings are present and perform checks
- class config_reader.UniqueKeyLoader(stream)¶
Special yaml loader with duplicate key checking.
Credits: ErichBSchulz, https://stackoverflow.com/questions/33490870/parsing-yaml-in-python-detect-duplicated-keys
Inheritance Diagram¶
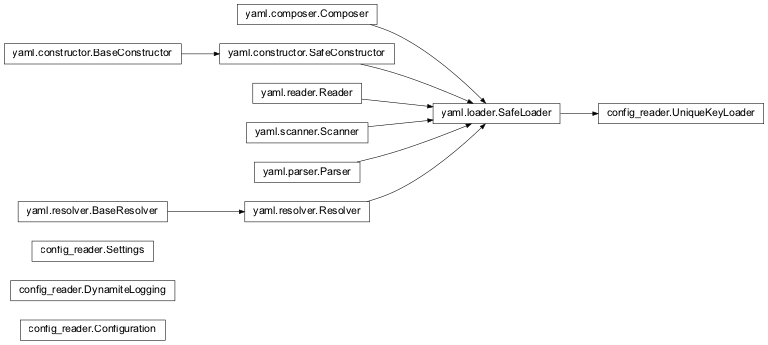