Parameter Space¶
Classes related to parameter spaces.
Algorithms to search through parameter space - a ParameterGenerator
- are defined here also.
ParameterGenerator
is a generic parent class, specific implementations are sub-classes of this.
API¶
Classes:
FullGrid
([par_space, parspace_settings])A full cartesian grid.
GridWalk
([par_space, parspace_settings])Walk after the current best fit
LegacyGridSearch
([par_space, parspace_settings])Search around all reasonable models
Parameter
([name, fixed, LaTeX, sformat, ...])Parameter of a model
ParameterGenerator
([par_space, ...])Abstract class for
ParameterGenerator
ParameterSpace
(system)A list of all
Parameter
objects in theModel
SpecificModels
([par_space, parspace_settings])Create specific models.
- class parameter_space.FullGrid(par_space=[], parspace_settings=None)¶
A full cartesian grid.
A full Cartesian grid in all free parameters, with bounds
lo/hi
and stepsizestep
. Warning: If several (>3) parameters are free, this will result in a large number of models. This parameter generator is generally not intended for production use.
- Parameters:
- par_space
dyn.parameter_space.ParameterSpace
object- parspace_settingsdict
Methods:
grid
([center, par, eps])Create the grid
specific_generate_method
(**kwargs)Generates new models
- grid(center=None, par=None, eps=1e-06)¶
Create the grid
Span the whole parameter grid defined by
self.par_space.par_generator_settings
attributes. IN GENERAL THIS WILL RESULT IN A LARGE NUMBER OF MODELS ADDED TO self.model_list! PRIMARILY THIS IS INTENDED FOR TESTING AND DEBUGGING. Clips parameter values to lo/hi attributes. If clipping violates the minstep attribute, the resulting model(s) will not be created. If the minstep attribute is missing, the step attribute will be used instead. Explicitly set minstep=0 to allow arbitrarily small steps down to eps (not recommended).
- Parameters:
- centerList of center coordinates. Must be in the same sequence as
the parameters in self.par_space. Mandatory argument.
- parInternal use only. Gives the parameter to start with. Set
automatically in the recursive process. The default is None.
- epsUsed for numerical comparison (relative tolerance), default 1e-6
- Returns:
- None. Sets self.model_list to the resulting models.
- Raises:
- ValueError if center is not specified or fixed parameters != center.
- specific_generate_method(**kwargs)¶
Generates new models
Span the whole parameter grid. The center of the grid walk is the parameter set with the smallest chi2 value, depending on the parameter space setting ‘which_chi2’.
- Parameters:
- None.
- Returns:
- None. self.model_list is the list of new models.
- Raises:
- None.
- class parameter_space.GridWalk(par_space=[], parspace_settings=None)¶
Walk after the current best fit
See docstrings for
specific_generate_method
andcheck_specific_stopping_critera
for full description.
- Parameters:
- par_space
dyn.parameter_space.ParameterSpace
object- parspace_settingsdict
Methods:
grid_walk
([center, par, eps])Walks the grid
specific_generate_method
(**kwargs)Generates new models
- grid_walk(center=None, par=None, eps=1e-06)¶
Walks the grid
Walks the grid defined by
self.par_space.par_generator_settings
attributes. Clips parameter values to lo/hi attributes. If clipping violates the minstep attribute, the resulting model(s) will not be created. If the minstep attribute is missing, the step attribute will be used instead. Explicitly set minstep=0 to allow arbitrarily small steps (not recommended).
- Parameters:
- centerList of center coordinates. Must be in the same sequence as
the parameters in self.par_space. Mandatory argument.
- parInternal use only. Gives the parameter to start with. Set
automatically in the recursive process. The default is None.
- epsUsed for numerical comparison (relative tolerance), default 1e-6
- Returns:
- None. Sets self.model_list to the resulting models.
- Raises:
- ValueError if center is not specified or fixed parameters != center.
- specific_generate_method(**kwargs)¶
Generates new models
The center of the grid walk is the parameter set with the smallest chi2 value, depending on the parameter space setting ‘which_chi2’.
- Parameters:
- None.
- Returns:
- None.
sets the list
self.model_list
of new models.- Raises:
- None.
- class parameter_space.LegacyGridSearch(par_space=[], parspace_settings=None)¶
Search around all reasonable models
This is the method used by previous code versions AKA schwpy. See docstrings for
specific_generate_method
andcheck_specific_stopping_critera
for full description.
- Parameters:
- par_space
dyn.parameter_space.ParameterSpace
object- parspace_settingsdict
Methods:
specific_generate_method
(**kwargs)Generates new models
- specific_generate_method(**kwargs)¶
Generates new models
Starts at the initial point. Start the iteration: (i) find all models with \(|\chi^2 - \chi_\mathrm{min}^2|\) within the specified threshold, (ii) for each model within the threshold, seed new models by independently take a step \(\pm 1\) of size
step
. If no new models are seeded at the end of an iteration, then divide all parameter stepsizes by two till their specifiedminstep
are reached.
- Parameters:
- None.
- Returns:
- None.
sets
self.model_list
is the list of new models.
- class parameter_space.Parameter(name=None, fixed=False, LaTeX=None, sformat=None, value=None, par_generator_settings=None, logarithmic=False)¶
Parameter of a model
- Parameters:
- namestring
the parameter name (specific components have specific parameter names)
- fixedBool
whether or not to fix this parameter during parameter searches
- LaTeXstring
a
`LaTeX
format string to use in plots- sformatstring
a format string for printing parameter values
- valuefloat
the value of this parameter in a model; the config file contains an initial value, this is updated during the parameter search; this value can be in log or linear units, depending on the config file
- par_generator_settingsdict
settings for the parameter generator
- logarithmicBool
whether or not this parameter is specified in log units
Methods:
get_par_value_from_raw_value
(raw_value)Get parameter value from the raw value
get_raw_value_from_par_value
(par_value)Get raw parameter value from parameter
update
(**kwargs)update the parameter
validate
()validate the parameter
Attributes:
getter method for par_value to be used like an attribute
- get_par_value_from_raw_value(raw_value)¶
Get parameter value from the raw value
In raw values, linearly-sized steps are taken during parameter searches. Currently there is only one possible raw transformation, going to log units. Future ones may include, e.g. isotropic transformations of viewing angles.
- Parameters:
- raw_valuefloat
the raw parameter value
- Returns:
- float
the parameter value
- get_raw_value_from_par_value(par_value)¶
Get raw parameter value from parameter
In raw values, linearly-sized steps are taken during parameter searches. Currently there is only one possible raw transformation, going to log units. Future ones may include, e.g. isotropic transformations of viewing angles.
- Parameters:
- par_valuefloat
the parameter value
- Returns:
- float
the raw parameter value
- property par_value¶
getter method for par_value to be used like an attribute
- update(**kwargs)¶
update the parameter
- validate()¶
validate the parameter
- class parameter_space.ParameterGenerator(par_space=[], parspace_settings=None, name=None)¶
Abstract class for
ParameterGenerator
ParameterGenerator
have methods to generate new sets of parameters to evaluate models based on existing models. This is an abstrct class, specific implementations should be implemented as child-classes (e.g.LegacyGridSearch
). Every implementation may have a methodcheck_specific_stopping_critera
and must have a methodspecific_generate_method
which define the stopping criteria and parameter generation algorithm for that implementation. These are exectuted in addition togeneric
methods, which are defined in this parentParameterGenerator
class.
- Parameters:
- par_space
dyn.parameter_space.ParameterSpace
object- parspace_settingsdict
parameter space settings
- namestring
the name of the particular ParameterGenerator sub-class
Methods:
add_model
([model, n_iter])Add a model
check generic stopping critera
checks specific stopping critera
Check stopping criteria
clip
(value, mini, maxi)clip to lo/hi
generate
([current_models, ...])Generate new parameter sets.
Placeholder.
- add_model(model=None, n_iter=0)¶
Add a model
Adds the model (a list of
Parameter
objects) to the tableself.current_models
. The datetime64 column is populated with the current timestamp numpy.datetime64(‘now’, ‘ms’). The ‘which_iter’ column is populated with the argument value n_iter.Note - here, model refers to a list of Parameter objects, not a
Model
object. TODO: clarify the naming confusion.
- Parameters:
- modelList of Parameter objects
- n_iterinteger
value to write in ‘which_iter’ column, optional. The default is 0.
- Returns:
- None.
- Raises:
- ValueError
If no or empty model is given.
- check_generic_stopping_critera()¶
check generic stopping critera
- check_specific_stopping_critera()¶
checks specific stopping critera
If the last iteration did not improve the chi2 by at least min_delta_chi2, then stop. May be overwritten or extended in each
ParameterGenerator
class.
- Returns:
- None
sets Bool to
self.status['min_delta_chi2_reached']
- check_stopping_critera()¶
Check stopping criteria
This is a wrapper which checks both the generic stopping criteria and also the
specific_stopping_critera
revelant for any particularParameterGenerator
used.
- clip(value, mini, maxi)¶
clip to lo/hi
Clips value to the interval [mini, maxi]. Similar to the numpy.clip() method. If mini==maxi, that value is returned.
- Parameters:
- valuenumeric value
- mininumeric value
- maxinumeric value
- Returns:
- min(max(mini, value), maxi)
- Raises:
- ValueError if mini > maxi
- generate(current_models=None, kw_specific_generate_method={})¶
Generate new parameter sets.
This is a wrapper method around the
specific_generate_method
of child generator classes. This wrapper does the following:
evaluates stopping criteria, and stops if necessary
runs the
specific_generate_method
of the child class, which updatesself.model_list
with a list of proposal modelsremoves previously run and invalid models from
self.model_list
converts parameters from raw_values to par_values
adds new, valid models to
current_models.table
update and return the status dictionary
- Parameters:
- current_modelsdynamite.AllModels
- kw_specific_generate_methoddict
keyword arguments passed to the specific_generate_method of the child class
- Returns:
- dict
- a status dictionary, with entries:
stop: bool, whether or not any stopping criteria are met
n_new_models: int
- and additional Bool entries for the indivdidual stopping criteria:
last_iter_added_no_new_models
n_max_mods_reached
n_max_iter_reached
plus any criteria specific to the child class
- specific_generate_method()¶
Placeholder.
This is a placeholder. Specific
ParameterGenerator
sub-classes should have their ownspecific_generate_method
methods.
- Parameters:
- None.
- Returns:
- None.
set
self.model_list
to be the list of newly generated models
- class parameter_space.ParameterSpace(system)¶
A list of all
Parameter
objects in theModel
- Parameters:
- systema
dyn.physical_system.System
objectMethods:
get_param_value_from_raw_value
(raw_value)Get parameter values from raw parameters
get_parameter_from_name
(name)Get a
Parameter
from the nameGet parset as row of an Astropy Table
get_raw_value_from_param_value
(par_val)Get raw parameter values from parameters
validate_parset
(parset)Validates a parameter set
Validates a parameter set
- get_param_value_from_raw_value(raw_value)¶
Get parameter values from raw parameters
In raw values, linearly-sized steps are taken during parameter searches. Currently there is only one possible raw transformation, going to log units. Future ones may include, e.g. isotropic transformations of viewing angles.
- Parameters:
- raw_valuelist of floats
list of raw parameter values for all models
- Returns:
- list of floats
list of parameter value
- get_parameter_from_name(name)¶
Get a
Parameter
from the name
- Parameters:
- namestring
- Returns:
dyn.parameter_space.Parameter
- get_parset()¶
Get parset as row of an Astropy Table
- Returns:
- parsetrow of an Astropy Table
Contains the values of the individual parameters.
- get_raw_value_from_param_value(par_val)¶
Get raw parameter values from parameters
In raw values, linearly-sized steps are taken during parameter searches. Currently there is only one possible raw transformation, going to log units. Future ones may include, e.g. isotropic transformations of viewing angles.
- Parameters:
- par_vallist of floats
list of parameter values for all models
- Returns:
- list of floats
list of raw parameter value
- validate_parset(parset)¶
Validates a parameter set
Validate the values of each component’s parameters by calling the individual components’ validate_parameter methods. Does the same for system parameters. Used by the parameter generators.
- Parameters:
- parsetlist of Parameter objects
- Returns:
- Bool
True if validation was successful, False otherwise
- validate_parspace()¶
Validates a parameter set
Validate the values of each component’s parameters by calling the individual components’ validate_parameter methods and check whether parameters are within the specified lo/hi bounds. Does the same for system parameters.
- Returns:
- None.
- Raises:
- ValueError
If checks fail due to various reasons.
- class parameter_space.SpecificModels(par_space=[], parspace_settings=None)¶
Create specific models.
Creates models with parameter values according to the entries in the lists
fixed_values
in a single iteration. If any parameter’sfixed_values
entry is missing, itsvalue
entry will be used.parspace_settings['generator_settings']['SpecificModels_mode']
determines how models are constructed:list
: selects parameter values element-wise. All parameters’fixed_values
lists must be of equal length (or zero length if their respectivevalue
entry is to be used).cartesian
: Cartesian product of fixed parameter values. The parameters’fixed_values
lists don’t need to be of equal length. May result in a large number of models.Note that this parameter generator ignores
step
,minstep
,lo
, andhigh
. Also,fixed
will be ignored iffixed_values
is specified.Further, all models are run in a single iteration and the optimality tolerances in the
stopping_criteria
section in the configuration file’sparameter_space_settings
will be ignored.
- Parameters:
- par_space
dyn.parameter_space.ParameterSpace
object- parspace_settingsdict
Methods:
The specific stopping critera
specific_generate_method
(**kwargs)Generates the specific models
- check_specific_stopping_critera()¶
The specific stopping critera
Will always stop after creating all specific models.
- Returns:
- None
sets
self.status['min_delta_chi2_reached']
toTrue
- specific_generate_method(**kwargs)¶
Generates the specific models
- Parameters:
- None.
- Returns:
- None.
sets
self.model_list
, the list of new models.
Inheritance Diagram¶
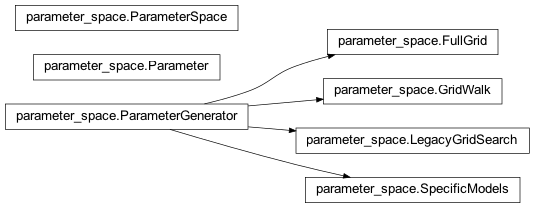