Model¶
Classes related to models, and collections of models (AllModels
).
API¶
Classes:
AllModels
([config, from_file])All models which have been run so far
Model
([config, parset, directory])A DYNAMITE model.
- class model.AllModels(config=None, from_file=True)¶
All models which have been run so far
The main attribute
self.table
is an Astropy table holding all models run so far.
- Parameters:
- configa
dyn.config_reader.Configuration
object- from_filebool
whether to create this object from a saved all_models.ecsv file
Methods:
convert_legacy_chi2_file
([legacy_filename])Convert legacy format of chi2 files
get_best_n_models
([n, which_chi2])Get the best n models or all but the n best models so far
get_best_n_models_idx
([n, which_chi2])Get the indices of the best n models so far
get_ml_of_original_orblib
(model_id)Get
ml
of model number model_id's original orblib
get_model_from_directory
(directory)Get the
Model
from a model directory
get_model_from_parset
(parset)Get the
Model
from a parset
get_model_from_row
(row_id)Get a
Model
given a table rowGet the model's velocity scaling factor
get_mods_within_chi2_thresh
([which_chi2, delta])Get models within or outside a delta threshold of the best
get_parset_from_row
(row_id)Get a parset set given a table row
get_row_from_model
([model])Get the table row for a
Model
make_best_models_table
([which_chi2, n, ...])Make a table of the best models and save it to disk
Make an empty Astropy table
read_legacy_chi2_file
(legacy_filename)Read the legacy AKA schwpy format of chi2 files
Read table from file
self.filename
Removes orbit libraries for 'bad' models.
Calculates kinmapchi2 for DYNAMITE legacy tables if possible.
save
()Save the all_models table
set_filename
(filename)Set the name (including path) for this model
all_models table update: fix incomplete models, add kinmapchi2.
- convert_legacy_chi2_file(legacy_filename=None)¶
Convert legacy format of chi2 files
legacy AKA schwpy format were likely called
`griddata/_chi2.cat
.
- Parameters:
- legacy_filename: string
the legacy_filename (probably griddata/_chi2.cat)
- get_best_n_models(n=10, which_chi2=None)¶
Get the best n models or all but the n best models so far
- Parameters:
- nint, optional
How many models to get. If negative, all models except the n best models will be returned. The default is 10.
- which_chi2str, optional
Which chi2 is used for determining the best models. If None, the setting from the configuration file will be used. The default is None.
- Returns:
- a new
astropy.table
object holding the best n models, sorted by- which_chi2
- Raises:
- ValueError
If which_chi2 is neither None nor a valid chi2 type.
- get_best_n_models_idx(n=10, which_chi2=None)¶
Get the indices of the best n models so far
- Parameters:
- which_chi2str, optional
Which chi2 is used for determining the best models. If None, the setting from the configuration file will be used. The default is None.
- Returns:
- list of int
indices in the all_models table of the n best model so far, sorted by which_chi2
- get_ml_of_original_orblib(model_id)¶
Get
ml
of model number model_id’s original orblibThe original
ml
is required to rescale orbit libraries for rescaled potentials. This method searchesself.table
, the all_models table.
- Parameters:
- model_idint
The
self.table
row index of the model.- Returns:
- ml_orblibfloat
the original
ml
- Raises:
- ValueError
If the
ml
parameter is not in the parameter space or the model’s orblib cannot be found.
- get_model_from_directory(directory)¶
Get the
Model
from a model directory
- Parameters:
- directorystr
The directory string needs to start with the output directory defined in
config.settings.io_settings['output_directory']
- Returns:
- moda
dyn.model.Model
object- Raises:
- ValueError
If the directory does not exist in the all_models table.
- get_model_from_parset(parset)¶
Get the
Model
from a parset
- Parameters:
- parsetlist
a list of
dyn.parspace.Parameter
objects- Returns:
- a
dyn.model.Model
object
- get_model_from_row(row_id)¶
Get a
Model
given a table row
- Parameters:
- row_idint
which row
- Returns:
- a
dyn.model.Model
object
- get_model_velocity_scaling_factor(model_id=None, model=None)¶
Get the model’s velocity scaling factor
Returns sqrt(model_ml/original_orblib_ml). The model can be either given by its row id in
self.table
or as aModel
object. Note that the parameters model_id and model are mutually exclusive.
- Parameters:
- model_idint compatible
The model’s row id in
self.table
.- modela
Model
object- Returns:
- scaling_factorfloat
The model’s velocity scaling factor sqrt(model_ml/original_orblib_ml).
- Raises:
- ValueError
If not exactly one of model_id and model are supplied.
- get_mods_within_chi2_thresh(which_chi2=None, delta=None)¶
Get models within or outside a delta threshold of the best
- Parameters:
- which_chi2str, optional
Which chi2 is used for determining the best models. If None, the setting from the configuration file will be used. The default is None.
- deltafloat, optional
The threshold value. Models with chi2 values differing from the opimum by at most delta will be returned. If None, models within 10% of the optimal value will be returned. If delta is negative, models that are NOT within a delta threshold of the best are returned. The default is to return models within 10% of the best.
- Returns:
- a new
astropy.table
object holding the ‘’delta-best’’ models- (if delta >= 0) or holding all but the ‘’delta-best’’ models
- (if delta < 0), respectively.
- Raises:
- ValueError
If which_chi2 is neither None nor a valid chi2 type.
- get_parset_from_row(row_id)¶
Get a parset set given a table row
- Parameters:
- row_idint
which row
- Returns:
- list
a list of
dyn.parspace.Parameter
objects
- get_row_from_model(model=None)¶
Get the table row for a
Model
- Parameters:
- modela
Model
object- Returns:
- row_idint
The
self.table
row index of model.- Raises:
- ValueError
If the model is not found in
self.table
.
- make_best_models_table(which_chi2=None, n=None, delta=None, filename=None)¶
Make a table of the best models and save it to disk
- Parameters:
- which_chi2str, optional
Which chi2 is used for determining the best models. If None, the setting from the configuration file will be used. The default is None.
- nint, optional
How many models to get. If None, n will be ignored. Default: if delta is specified, the default is none; if delta is None, the default is 10.
- deltafloat, optional
The threshold value. Models with chi2 values differing from the opimum by at most delta will be returned. If None, delta will be ignored. The default is None.
- filenamestr, optional
File name of the best models table. The file is written into the output directory specified in the config file. If None, the name is the same as for the all models table but with ‘_best’ added to the base file name. If the file already exists, a warning is logged, the existing file is backed up (‘_backup’ added), and then overwritten. The default is None.
- Returns:
- int
The number of models in the best models table.
- Raises:
- ValueError
If both n and delta are specified (i.e., both are not None).
- make_empty_table()¶
Make an empty Astropy table
- Returns:
- None
sets
self.table
- read_legacy_chi2_file(legacy_filename)¶
Read the legacy AKA schwpy format of chi2 files
Taken from schw_basics.py, reads in legacy files named similar to griddata/_chi2.cat
- Parameters:
- legacy_filename: string
the legacy_filename (probably griddata/_chi2.cat)
- read_model_table()¶
Read table from file
self.filename
- Returns:
- None
sets
self.table
- remove_unused_orblibs()¶
Removes orbit libraries for ‘bad’ models.
Frees disk space by deleting data, keeping only model data required by the
beta_plot
andmass_plot
plotting routines. Keeps data of models with (kin)chi2 values less than or equal tosqrt(2 * number of kinematic observations) * min(chi2)
, but at least 3 models. Will mark a deleted orbit library withorblib_done=False
andweights_done=False
in the all_models table. If an orblib cannot be deleted because it is used by another model, only the nnls data will be deleted, which is marked byweights_done=False
in the all_models table.
- Returns:
- bool
True
if data deletion has been attempted,False
if no data to delete could be identified.
- retrofit_kinmapchi2()¶
Calculates kinmapchi2 for DYNAMITE legacy tables if possible.
- Returns:
- None.
updates
self.table
- save()¶
Save the all_models table
- set_filename(filename)¶
Set the name (including path) for this model
- Parameters:
- filenamestring
name for this model
- Returns:
- None
sets
self.filename
- update_model_table()¶
all_models table update: fix incomplete models, add kinmapchi2.
Dealing with incomplete models: Models with all_done==False but an existing model_done_staging.ecsv will be updated in the table and the staging file will be deleted. Models with all_done==False and no existing orblib will be deleted from the table and their model directory will be deleted, too. The configuration setting reattempt_failures determines how partially completed models with all_done==False but existing orblibs are treated: If reattempt_failures==True, their orblib_done will be set to True and later the ModelIterator will execute the weight solving based on the existing orblibs. If reattempt_failures==False, the model and its directory will be deleted. Note that orbit libraries on disk will not be deleted as they may be in use by other models.
Up to DYNAMITE 3.0 there was no kinmapchi2 column in the all_models table. If possible (data exists on disk), calculate and add the values, otherwise set to np.nan.
- Returns:
- None
sets
self.table
- class model.Model(config=None, parset=None, directory=None)¶
A DYNAMITE model.
The model can be run by running the methods (i) get_orblib, (ii) get_weights and (iii) (in the future) do_orbit_colouring. Running each of these methods will return the appropriate object, e.g. model.get_orblib() –> returns an OrbitLibrary object model.get_weights(…) –> returns a WeightSolver object
- Parameters:
- configa
dyn.config_reader.Configuration
object- parsetrow of an Astropy Table
contains the values of the potential parameters for this model
- directorystr
The model directory name (without path). If None or not specified, the all_models_file will be searched for the directory name. If the all_models file does not exist, the model directory will be set to
orblib_000_000/ml{ml}
.- Returns:
- Nothing returned. Attributes holding outputs are are added to the
- object when methods are run.
Methods:
check_parset
(parspace, parset)Validate a parset
create_model_directory
(path)make a directory if it does not yet exist
get the name of this model's output directory
Make the orbit library
get_weights
([orblib])Get the orbital weights
setup directories
Validate the content of the config file against the model's config file
- check_parset(parspace, parset)¶
Validate a parset
Given parameter values in parset, the validate_parspace method of the parameter space is executed. If a parameter exists in parspace but not in parset, a warning will be issued and the parameter will remain unchanged. If parset tries to set the value of a parameter not existing in parspace, an exception will be raised. Validating relies on exceptions raised by validate_parspace.
- Parameters:
- parspace
dyn.parameter_space.ParameterSpace
A list of parameter objects.
- parsetrow of an Astropy Table
Contains parameter values to be checked against the settings in parspace.
- Returns:
- None.
- Raises:
- ValueError
If at least one parameter in parset is unknown to parspace.
- create_model_directory(path)¶
make a directory if it does not yet exist
- Parameters:
- pathstring
directory path to make
- get_model_directory()¶
get the name of this model’s output directory
- Returns:
- string
- get_orblib()¶
Make the orbit library
- Returns:
- a
dyn.orblib.OrbitLibrary
object
- get_weights(orblib=None)¶
Get the orbital weights
- Parameters:
- orbliba
dyn.orblib.OrbitLibrary
object- Returns:
- a
dyn.weight_solver.WeightSolver
object
- setup_directories()¶
setup directories
- validate_config_file()¶
Validate the content of the config file against the model’s config file
Upon solving a model, DYNAMITE creates a backup of the config file in the model directory. Instantiating a model later (e.g., for plotting) using a config file that is incompatible with the one used to create the model can lead to problems (e.g., differently sized orbit library). This method validates the “global” config file against the one in the model directory (if existing).
Differing config files may be ok and intended (e.g., due to expanding the parameter space). Therefore, the main purpose of this method is to add warnings to the log to alert the user.
- Returns:
- bool
False
if a config file backup is successfully found in the model directory and it differs from the “global” config file.True
otherwise (no config file backup could be identified or the config file backup is identical to the global config file).- Raises:
- FileNotFoundError
If the “global” config file cannot be found.
Inheritance Diagram¶
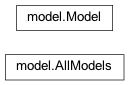