Physical System¶
Classes related to the physical System
being modelled, which consists of Components
.
API¶
Classes:
AxisymmetricVisibleComponent
(**kwds)
BarDiskComponent
([mge_pot, mge_lum, ...])Rotating triaxial component with a MGE projected density (i.e. a bar).
BarDiskComponentAngles
([mge_pot, mge_lum, ...])Rotating triaxial component with a MGE projected density (i.e. a bar), with viewing angles specified.
Component
([name, visible, ...])A component of the physical system
DarkComponent
([density])Any dark component of the sytem, with no observed MGE or kinemtics
GeneralisedNFW
(**kwds)A GeneralisedNFW halo
Hernquist
(**kwds)A Hernquist sphere
NFW
(**kwds)An NFW halo
NFW_m200_c
(**kwds)An NFW halo with m200-c relation from Dutton & Maccio 14
Plummer
(**kwds)A Plummer sphere
System
(*args)The physical system being modelled
TriaxialCoredLogPotential
(**kwds)A TriaxialCoredLogPotential
TriaxialVisibleComponent
(**kwds)Triaxial component with a MGE projected density
VisibleComponent
([mge_pot, mge_lum])Any visible component of the sytem, with an MGE
- class physical_system.AxisymmetricVisibleComponent(**kwds)¶
Methods:
validate
()Validate the component
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.BarDiskComponent(mge_pot=None, mge_lum=None, disk_pot=None, disk_lum=None, **kwds)¶
Rotating triaxial component with a MGE projected density (i.e. a bar)
Note: all bar components are constrained to have the same omega.
- class physical_system.BarDiskComponentAngles(mge_pot=None, mge_lum=None, disk_pot=None, disk_lum=None, **kwds)¶
Rotating triaxial component with a MGE projected density (i.e. a bar), with viewing angles specified.
Note: all bar components are constrained to have the same omega.
Methods:
validate_parset
(par)Validate the p, q, u parameters
- validate_parset(par)¶
Validate the p, q, u parameters
Validates the triaxial component’s p, q, u parameters. Requires self.qobs to be set. A parameter set is valid if the resulting (theta, psi, phi) are not np.nan.
- Parameters:
- pardict
{ “p”:val, … } where “p” are the component’s parameters and val are their respective values
- Returns:
- bool
True if the parameter set is valid, False otherwise
- class physical_system.Component(name=None, visible=None, contributes_to_potential=None, symmetry=None, kinematic_data=[], population_data=[], parameters=[])¶
A component of the physical system
e.g. the stellar component, black hole, or dark halo of a galaxy
- Parameters:
- namestring
a short but descriptive name of the component
- visibleBool
whether this is visible <–> whether it has an associated MGE
- contributes_to_potentialBool
whether this contributes_to_potential not currently used
- symmetrystring
one of ‘spherical’, ‘axisymm’, or ‘triax’ not currently used
- kinematic_datalist
a list of
dyn.kinemtics.Kinematic
data for this component- parameterslist
a list of
dyn.parameter_space.Parameter
objects for this component- population_datalist
a list of
dyn.populations.Population
data for this component not currently usedMethods:
Get a parameter using its (unsuffixed) name.
get_parname
(par)Strip the component name suffix from the parameter name.
validate
([par])Validate the component
validate_parset
(par)Validates the component's parameter values.
- get_par_by_name(n)¶
Get a parameter using its (unsuffixed) name.
- Parameters:
- nstr
The parameter name (without the component name suffix)
- Returns:
- pa
dyn.parameter_space.Parameter
objectThe parameter in question.
- get_parname(par)¶
Strip the component name suffix from the parameter name.
- Parameters:
- parstr
The full parameter name “parameter-component”.
- Returns:
- pure_parnamestr
The parameter name without the component name suffix.
- validate(par=None)¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- validate_parset(par)¶
Validates the component’s parameter values.
Kept separate from the validate method to facilitate easy calling from the parameter generator class. This is a placeholder method which returns True if all parameters are non-negative, except for logarithmic parameters which are not checked. Specific validation should be implemented for each component subclass.
- Parameters:
- pardict
{ “p”:val, … } where “p” are the component’s parameters and val are their respective raw values
- Returns:
- isvalidbool
True if the parameter set is valid, False otherwise
- class physical_system.DarkComponent(density=None, **kwds)¶
Any dark component of the sytem, with no observed MGE or kinemtics
This is an abstract layer and none of the attributes/methods are currently used.
Methods:
get_dh_legacy_strings
(parset)Generates and returns two strings needed for the legacy Fortran files.
- get_dh_legacy_strings(parset)¶
Generates and returns two strings needed for the legacy Fortran files.
This method only applies to dark halo components.
- Parameters:
- parsetastropy table row
Holds the parameter set.
- Returns:
- specsstr
A string with the legacy code and the number of parameters, space separated.
- par_valsstr
The parameter values in the sequence legacy Fortran expects them, space separated.
- class physical_system.GeneralisedNFW(**kwds)¶
A GeneralisedNFW halo
from Zhao (1996) Defined with parameters: concentration [R200/NFW scale length], Mvir [Msol], inner_log_slope []
Methods:
validate
()Validate the component
validate_parset
(par)Validates the GeneralisedNFW's parameters.
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- validate_parset(par)¶
Validates the GeneralisedNFW’s parameters.
Requires c and Mvir >0, and gam leq 1
- Parameters:
- pardict
{ “p”:val, … } where “p” are the component’s parameters and val are their respective values
- Returns:
- bool
True if the parameter set is valid, False otherwise
- class physical_system.Hernquist(**kwds)¶
A Hernquist sphere
Defined with parameters: rhoc [central density, Msun/km^3] and rc [scale length, km]
Methods:
validate
()Validate the component
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.NFW(**kwds)¶
An NFW halo
Defined with parameters: c [concentration, R200/scale] and f [dm-fraction, M200/total-stellar-mass]
Methods:
validate
()Validate the component
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.NFW_m200_c(**kwds)¶
An NFW halo with m200-c relation from Dutton & Maccio 14
The relation: log10(c200) = 0.905 - 0.101 * log10(M200/(1e12/h)). Component defined with parameter f [dm-fraction, M200/total-stellar-mass]
Methods:
get_c200
(system, parset)Calculates and returns c200 (see Dutton & Maccio 2014).
get_dh_legacy_strings
(parset, system)Generates and returns two strings needed for the legacy Fortran files.
validate
()Validate the component
- get_c200(system, parset)¶
Calculates and returns c200 (see Dutton & Maccio 2014).
- Parameters:
- systema
dyn.physical_system.System
object- parsetastropy table row
Must contain dark matter fraction f-{self.name} and ml
- Returns:
- float
c200
- get_dh_legacy_strings(parset, system)¶
Generates and returns two strings needed for the legacy Fortran files.
This method overrides the parent class’ method because for legacy Fortran purposes, NFW_m200_c has two parameters. Note that NFW_m200_c needs an addiional parameter
system
.
- Parameters:
- parsetastropy table row
Holds the parameter set.
- Returns:
- specsstr
A string with the legacy code and the number of parameters, space separated.
- par_valsstr
The parameter values in the sequence legacy Fortran expects them, space separated.
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.Plummer(**kwds)¶
A Plummer sphere
Defined with parameters: M [mass, Msol] and a [scale length, arcsec]
Methods:
validate
()Validate the component
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.System(*args)¶
The physical system being modelled
e.g. system is a galaxy. A system is composed of
Components
e.g. the galaxy is composed of stars, black hole, dark matter halo. This object is automatically created when the configuration file is read.Methods:
add_component
(cmp)add a component to the system
Get all components which are rotating MGEs (i.e. bars).
Get all components which are Dark
Get all Dark components which are not plummer
Loop over all components, extract their kinemtics into a list.
Get all components which contain MGEs
get_component_from_class
(cmp_class)
get_component_from_name
(cmp_name)Get a parameter using its name.
Return the unique rotating bar component (raises an error if there are zero or multiple such components)
Return the unique non-bar MGE component (raises an error if there are zero or multiple such components)
Check if the system contains at least one bar component and at least one disk component.
Check if the system is a bar-disk with phi, psi, theta specified directly in the configuration file.
validate
()Validate the system
validate_parset
(par)Validates the system's parameter values
- add_component(cmp)¶
add a component to the system
- Parameters:
- cmpa
dyn.physical_system.Component
object- Returns:
- None
updated the system componenent attributes
- get_all_bar_components()¶
Get all components which are rotating MGEs (i.e. bars)
- Returns:
- list
a list of Component objects, keeping only the rotating MGE components
- get_all_dark_components()¶
Get all components which are Dark
- Returns:
- list
a list of Component objects, keeping only the dark components
- get_all_dark_non_plummer_components()¶
Get all Dark components which are not plummer
Useful in legacy orbit libraries for finding the dark halo component. For legacy models, the black hole is always a plummer, so any Dark but non plummer components must represent the dark halo.
- Returns:
- list
a list of Component objects, keeping only the dark components
- get_all_kinematic_data()¶
Loop over all components, extract their kinemtics into a list.
- Returns:
- list
all_kinematics in a list
- get_all_mge_components()¶
Get all components which contain MGEs
- Returns:
- list
a list of Component objects
- get_component_from_class(cmp_class)¶
- Parameters:
- cmp_classstring
name of the component type/class
- Returns:
- a
dyn.physical_system.Component
object- Raises:
- ValueErrorif there are more than one component of the same class.
# TODO: remove this limit, e.g. if we had two MGE-based components one for stars, one for gas
- get_component_from_name(cmp_name)¶
- Parameters:
- cmp_namestring
component name (as specified in the congi file)
- Returns:
- a
dyn.physical_system.Component
object
- get_par_by_name(n)¶
Get a parameter using its name.
- Parameters:
- nstr
The parameter name.
- Returns:
- pa
dyn.parameter_space.Parameter
objectThe parameter in question.
- get_unique_bar_component()¶
Return the unique rotating bar component (raises an error if there are zero or multiple such components)
- Returns:
- a
dyn.physical_system.BarDiskComponent
object
- get_unique_triaxial_visible_component()¶
Return the unique non-bar MGE component (raises an error if there are zero or multiple such components)
- Returns:
- a
dyn.physical_system.TriaxialVisibleComponent
object
- is_bar_disk_system()¶
Check if the system contains at least one bar component and at least one disk component.
- Returns:
- isbardiskBool
System contains a bar and a disk.
- is_bar_disk_system_with_angles()¶
Check if the system is a bar-disk with phi, psi, theta specified directly in the configuration file.
- Returns:
- hasanglesBool
System is specified by angles.
- validate()¶
Validate the system
Ensures the System has the required attributes: at least one component, no duplicate component names, and the ml parameter, and that the sformat string for the ml parameter is set. Also validates the parameter generator settings’ minstep value for ml if it is a non-fixed parameter.
- Returns:
- None.
- Raises:
- ValueErrorif required attributes or components are missing, or if
there is no ml parameter
- validate_parset(par)¶
Validates the system’s parameter values
Kept separate from the validate method to facilitate easy calling from the
ParameterGenerator
class. Returns True if all parameters are non-negative, except for logarithmic parameters which are not checked.
- Parameters:
- pardict
{ “p”:val, … } where “p” are the system’s parameters and val are their respective raw values
- Returns:
- isvalidbool
True if the parameter set is valid, False otherwise
- class physical_system.TriaxialCoredLogPotential(**kwds)¶
A TriaxialCoredLogPotential
see e.g. Binney & Tremaine second edition p.171 Defined with parameters: p [B/A], q [C/A], Rc [core radius, kpc], Vc [asympt. circular velovity, km/s]
Methods:
validate
()Validate the component
- validate()¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
- class physical_system.TriaxialVisibleComponent(**kwds)¶
Triaxial component with a MGE projected density
Has parameters (p,q,u) = (b/a, c/a, sigma_obs/sigma_intrinsic) used for deprojecting the MGE. A given (p,q,u) correspond to a fixed set of viewing angles for the triaxial ellipsoid.
Methods:
find_grid_of_valid_pqu
([n_grid])Find valid values of the parameters (p,q,u)
suggest_parameter_values
([target_u])Suggest valid values of the parameters (p,q,u)
triax_pqu2tpp
(p, q, u)transform axis ratios to viewing angles
validate
()Validate the TriaxialVisibleComponent
validate_parset
(par)Validate the p, q, u parameters
- find_grid_of_valid_pqu(n_grid=200)¶
Find valid values of the parameters (p,q,u)
Creates a grid of all values of 0<(p,q,u)<=1, and finds those which have a valid deprojection subject to the fulfilment of all three criteria: 1. 0 < q <= p <=1 2. max(q/qobs, p) < u 3. u< min(p/qobs, 1) where qobs is the smallest value of q for the MGE.
- Parameters:
- n_gridint
grid size used for p,q,u
- Returns:
- (p,q,u), valid
3d grids of p,q,u values, and boolen array valid which is True for valid values
- suggest_parameter_values(target_u=0.9)¶
Suggest valid values of the parameters (p,q,u)
Find valid values using the mehtod find_grid_of_valid_pqu. Then for each of (p,q,u), we suggest values: - lo/hi : the min/max of all valid values - value : u=target_u, and p/q = mean of all valid p/q values where u is close to target value - step/minstep : a fifth/twentieth of the range of valid values
- Parameters:
- target_ufloat
Desired value of the parameter u
- Returns:
- string
text to print out suggesting quantities for (p,q,u)
- triax_pqu2tpp(p, q, u)¶
transform axis ratios to viewing angles
transfer (p, q, u) to the three viewing angles (theta, psi, phi) with known flatting self.qobs. Taken from schw_basics, same as in vdB et al. 2008, MNRAS 385,2,647 We should possibly revisit the expressions later
- validate()¶
Validate the TriaxialVisibleComponent
Validates parameter names and sets self.qobs (minimal flattening from mge data).
- Returns:
- None.
- validate_parset(par)¶
Validate the p, q, u parameters
Validates the triaxial component’s p, q, u parameters. Requires self.qobs to be set. A parameter set is valid if the resulting (theta, psi, phi) are not np.nan.
- Parameters:
- pardict
{ “p”:val, … } where “p” are the component’s parameters and val are their respective values
- Returns:
- bool
True if the parameter set is valid, False otherwise
- class physical_system.VisibleComponent(mge_pot=None, mge_lum=None, **kwds)¶
Any visible component of the sytem, with an MGE
- Parameters:
- mge_pota
dyn.mges.MGE
objectdescribing the (projected) surface-mass density
- mge_luma
dyn.mges.MGE
objectdescribing the (projected) surface-luminosity density
Methods:
get_M_stars_tot
(distance, parset)Calculates and returns the total stellar mass via the mge.
validate
(**kwds)Validate the component
- get_M_stars_tot(distance, parset)¶
Calculates and returns the total stellar mass via the mge.
- Parameters:
- distancefloat
Distance of the system in MPc
- parsetastropy table row
must contain mass-to-light ratio ml
- Returns:
- float
Total stellar mass
- validate(**kwds)¶
Validate the component
Ensure it has the required attributes and parameters. Also validates the parameter generator settings’ minstep value for non-fixed parameters.
- Parameters:
- para list with parameter names. Mandatory.
- Returns:
- None.
- Raises:
- ValueErrorif a required attribute is missing or the required
parameters do not exist
Inheritance Diagram¶
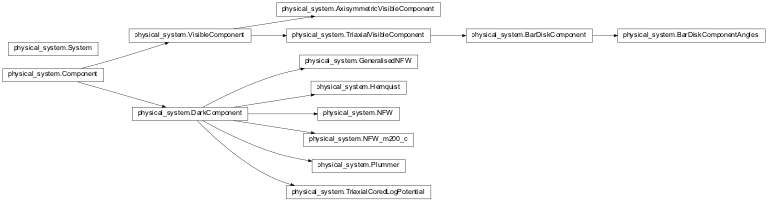